Google font not loading on NextJS website
Set up google fonts on my NextJS app that simply refused to work. Here’s how I fixed it.
Aug 7, 2024
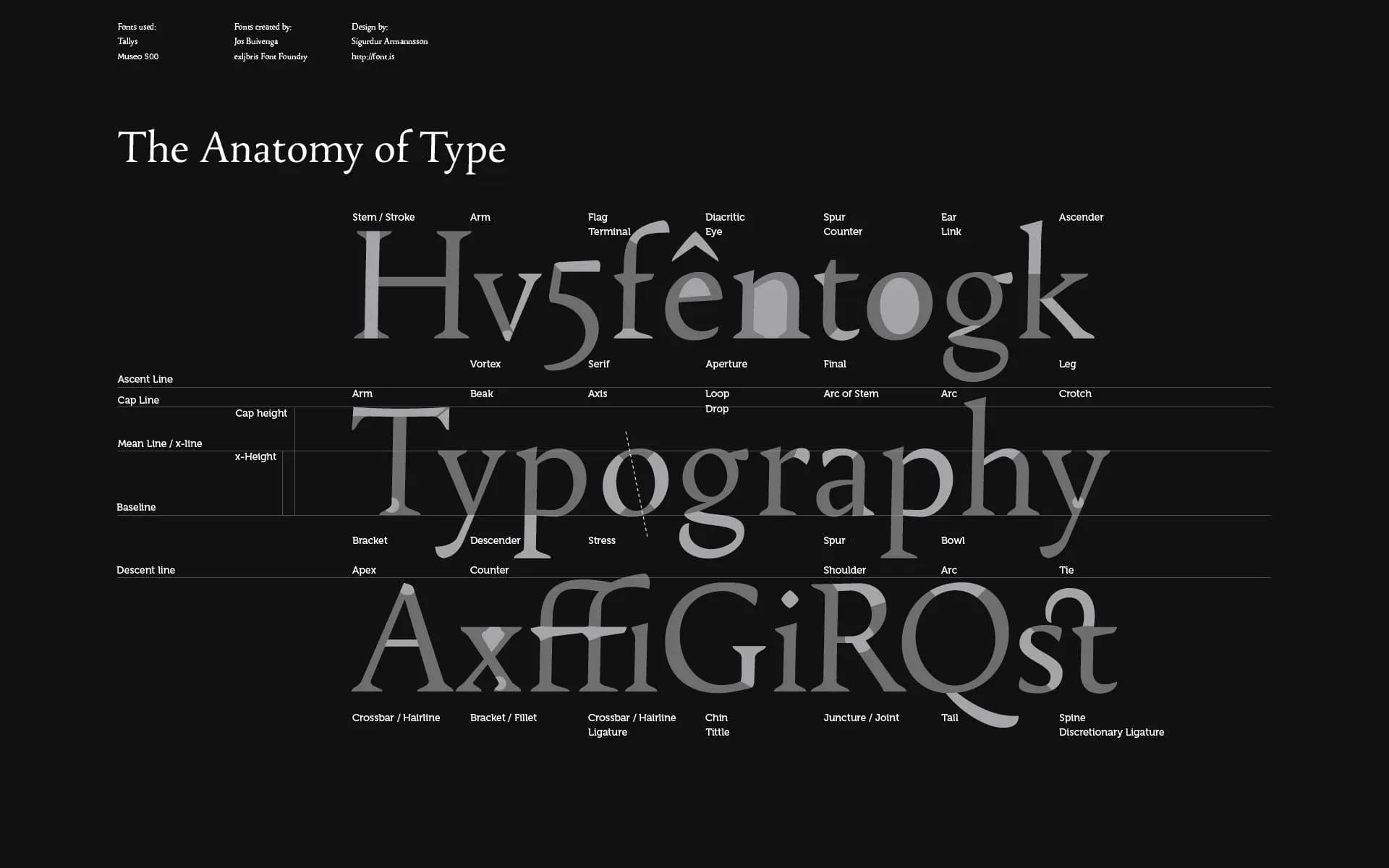
On a NextJS website I was building, I did everything that was required to link up my website with google fonts using next/fonts in my layout file since I wanted whatever logic I setup to reflect on my entire website.
Everything was set properly and yet, the fonts refused to work.
Since I was using two fonts, Montserrat and Roboto, I ended up having two solutions that solved the problem for each font.
- Roboto wasn’t loading because I didn’t add the weight property.
- Montserrat wasn’t loading because although I added the subset of ‘latin’, it wasn’t sufficient. Had to add all other subsets before it started working. Very curious solution but it worked. I can only come to the conclusion that latin as a subset wasn’t sufficient for whatever reason.
And that’s that.
If you set up your fonts properly and it still doesn’t work, try both of these steps.
And that’s all from me.
ADDITION
Oh yes, here’s how to setup the font on the layout.js file:
First, you import the font’s from next/font/google
import { Poppins, Paytone_One } from "next/font/google";
Next, you create a variable to hold both:
const poppins = Poppins({
subsets: ["latin"],
weight: ['100', '200', '300', '400', '500', '600', '700', '800', '900'],
variable: '--font-poppins' });
const paytone = Paytone_One({
subsets: ["latin"],
weight: ['400'],
variable: '--font-paytone' });
Then body of your export statement should look like this:
<body className={`${poppins.variable` ${paytone.variable}'}>
Then on the tailwind.config.js file:
fontFamily: {
paragraph: ['var(--font-poppins)'],
heading: ['var(--font-paytone)'],
},
And to make use of the font, you can use the global.css
h1, h2, h3, h4, h5, h6, a {
font-family: var(--font-paytone);
}
p, label {
font-family: var(--font-poppins);
}
And that should be that.